Testing Ruby Code with RSpec-mocks: Examples and Tips
Last updated: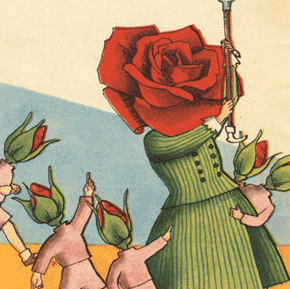
Table of Contents
Custom block to define what a double will return
When none of the predefined ways to define what your double will return (once()
, twice()
and so on) work for you, RSpec lets you specify a block to define exactly what you want a double to return.
For example, I want to make a double that will receive a method called :take!
exactly four times. In the first three calls it should return 1
and on the fourth call it should raise an Exception
:
it 'contrived example' do
my_double = double()
# create a counter outside so you can keep track
# of how many times the method was called
count = 0
expect(my_double).to receive(':take!').exactly(4).times {
count += 1
if count == 4
raise Exception.new("Ka-boom!")
else
1
end
}
# rest of spec code...
# ...
end
References: