Yii Routes and URL Generation: Quick Explanation and Examples
Last updated: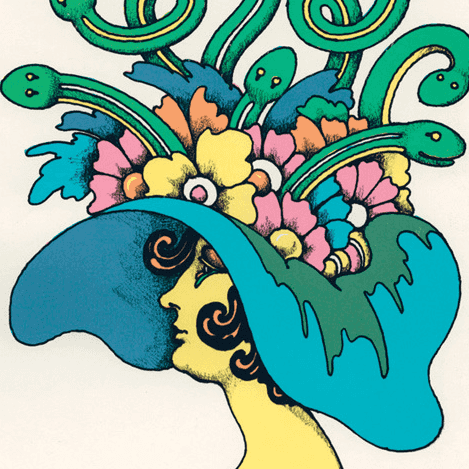
Routing is very important on any web framework and this is also true on Yii. Apart from configuring the actual routes in your main.php
config file, you'll probably use Yii::app()->createUrl()
many, many times on your app.
Here is a small reminder on the basic stuff you need to know about routes on Yii.
Sample main.php
config file showing routes
<?php
// previous lines ignored
'urlManager' => array(
'urlFormat' => 'path',
'showScriptName' => false,
'rules' => array(
// specific rules
// route => controller/action
'departments/<id:\d+>/add_employee>' => 'departments/addEmployee',
'employees/<id:\d+>/add_to_department/<department_name:\w+>' => 'employees/addToDepartment',
// catch-all rules
// this file is read in order so it only matches if no other rules
// above have matched
'<controller:\w+>' => '<controller>/index',
'<controller:\w+>/<id:\d+>' => '<controller>/view',
'<controller:\w+>/<id:\d+>/<action:\w+>' => '<controller>/<action>'
)
),
URL to Controller Action
The specific rules mean the following to Yii:
If you receive a request that matches the left-hand side, this is what Yii will do:
'departments/5/add_employee'
will cause functionactionAddEmployee(5)
to will be called inDepartmentsController
, so you should have a function like this:
<?php class DepartmentsController extends Controller{ // previous lines ignored public function actionAddEmployee($id){ // stuff... } // other methods
'employees/15/add_to_department/sales'
will cause functionactionAddToDepartment(15,'sales')
to be called inEmployeesController
or, in other words:
<?php class EmployeesController extends Controller{ // previous lines ignored public function actionAddToDepartment($id,$department_name){ // stuff } // other methods
This is how you generate a method call from a url. But how do you generate an url from a controller action?
Controller Action to URL
To generate a url from a controller/action route (for example, for links) you'll probably use Yii::app()->createUrl($route, $params). It takes a route (String) and parameters (Array), like this:
<?php
$url = Yii::app()->createUrl('departments/addEmployee', array('id' => 10));
// $url equals "/departments/10/add_employee"
$url2 = Yii::app()->createUrl('employees/addToDepartment',array('id' => 34, 'department_name' => 'marketing'));
// $url2 equals "/employees/34/add_to_department/marketing"