Scala Imports: Quick Intro and Examples
Last updated: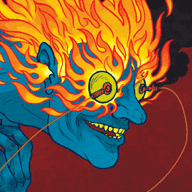
Imports (available in other languages via constructs like require, include or load), help us keep our code modular and weakly coupled with other parts of our application.
In Scala, as with many other languages, one can (should) split their code into modules and then import them into other modules, so that only their public interface is visible to them.
One key idea here is that of packages, which is the way modules are known in the Java (and therefore in the Scala) world.
You can use code from other packages if you a) fully qualify them or b) import them into the current scope. In Scala, you can do anything you are able to in Java and plus a few other tricks:
Simplest use case
It's just like Java:
import path.to.my.package.MyClass
val p = new MyClass
Import all classes in a package
Similar to using the star (*) in Java:
import path.to.my.package._
//all classes in the imported package can now be used
//supposing classes ClassA and ClassB were members of that package:
val a = new ClassA
val b = new ClassB
Importing multiple Classes
import path.to.my.package.{
ClassA, ClassB
}
val a = new ClassA
val b = new ClassB
Aliases
If you have used Python before, chances are you liked renaming your imports with aliases to make your code easier to read (e.g. import numpy as np
) . You can do that in Scala too:
import path.to.my.package.{
SomeClassWithAVeryLongName => ThisClass,
SomeOtherClassYouWantToRename => ThatClass
}
val foo = new ThisClass
val bar = new ThatClass
Importing an Object into scope
You can also import objects into scope. This can be used to import companion objects (just containers for static methods on a class) or regular objects:
import path.to.my.package.HelperObject._
//you can now use all methods in object HelperObject
val a = helperMethod("foo","bar")
Imports can also be declared inside defined scopes!
For example, if you only want a set of methods to be accessible inside a function, you can do so:
def timesPi(num:Int):Double = {
import math._
// methods (and constants) are only available inside this function!
num * Pi
}