Pandas Dataframe: Replace Examples
Last updated:Table of Contents
View examples on this notebook
Replace value anywhere
use
inplace=True
to mutate the dataframe itself
This is the simplest possible example.
Usedf.replace([v1,v2], v3)
to replace all occurrences of v1
and v2
with v3
import pandas as pd
df = pd.DataFrame({
'name':['john','mary','paul'],
'age':[30,25,40],
'city':['new york','los angeles','london']
})
df.replace([25],40)
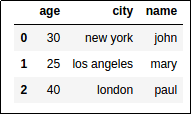
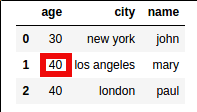
Replace with dict
Use df.replace{from1:to1, from2:to2, ...}
import pandas as pd
df = pd.DataFrame({
'name':['john','mary','paul'],
'age':[30,25,40],
'city':['new york','los angeles','london']
})
df.replace({
25:26,
'john':'johnny'
})
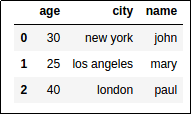
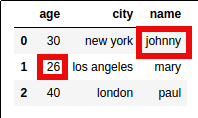
Replace with regex
Use df.replace(pattern, replacement, regex=True)
import pandas as pd
df = pd.DataFrame({
'name':['john','mary','paul'],
'age':[30,25,40],
'city':['new york','los angeles','london']
})
df.replace('jo.+','FOO',regex=True)
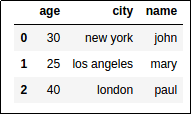
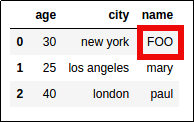
Replace in single column
Use df.replace({colname:{from:to}})
df = pd.DataFrame({
'name':['john','mary','paul'],
'num_children':[0,4,5],
'num_pets':[0,1,2]
})
# replace 0 with 1 in column "num_pets" only!
df.replace({'num_pets':{0:1}})
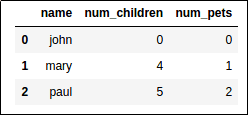
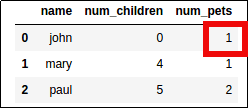
replacements in a specific column only.